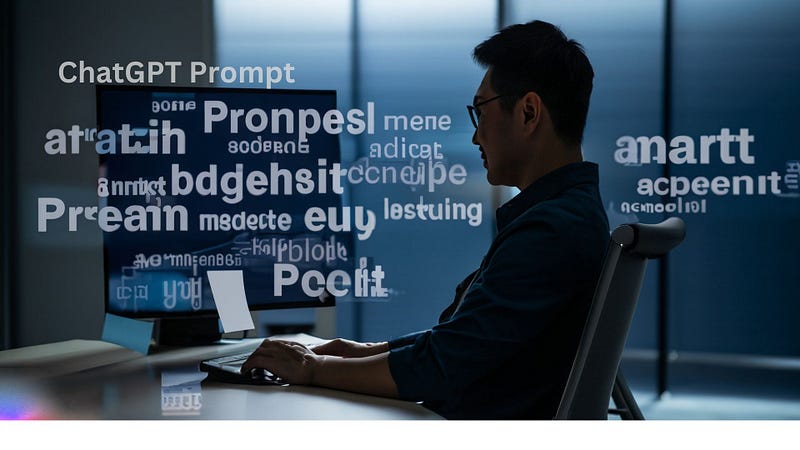
Prompt engineering is essential for optimizing behavior and output of AI systems like ChatGPT. Creating effective prompts is challenging and time-consuming, especially when tailoring them for different roles/persona. However, we can utilize ChatGPT’s capabilities to generate prompts for us.
By empowering ChatGPT with custom knowledge on crafting effective prompts, we enable it to learn the skill of generating prompts tailored to various roles. To impart this custom knowledge, we leverage a collection of websites that provide good instances of well-crafted system prompts.
In this blog post, we will explore using Python, ChatGPT, and the LangChain module to generate role-specific prompt phrases. LangChain is a versatile tool that enables the conversion of external sources into document objects. Converted document objects can then be indexed in databases like Chroma, enabling fast and efficient information retrieval. This integration allows AI systems such as ChatGPT to access a broad spectrum of knowledge sources.
Setting up the Environment
Below code snippet demonstrates the necessary steps to extract additional data from websites, create embeddings, utilize Chroma vector store, load documents from the web, and persist the processed instance. These steps lay the foundation for generating system prompt phrases using the extracted data.
# intalling the necessary libraries in Jupyter
!pip install tiktoken
!pip install openai
!pip install chromadb
!pip install langchain
!pip install nest_asyncio
from langchain.embeddings.openai import OpenAIEmbeddings
from langchain.vectorstores import Chroma
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.llms import OpenAI
from langchain.chains import RetrievalQA
from langchain.chat_models import ChatOpenAI
from langchain.document_loaders import WebBaseLoader
from langchain.prompts import PromptTemplate
import nest_asyncio
nest_asyncio.apply()
# sample website with good system prompts
tgt_sites = ['https://github.com/f/awesome-chatgpt-prompts',
'https://www.greataiprompts.com/prompts/best-system-prompts-for-chatgpt/',
'https://stackdiary.com/chatgpt/role-based-prompts/']
def add_documents(loader, instance):
documents = loader.load()
text_splitter = RecursiveCharacterTextSplitter(chunk_size=1000, chunk_overlap=100, separators= ["\n\n", "\n", ".", ";", ",", " ", ""])
texts = text_splitter.split_documents(documents)
instance.add_documents(texts)
embeddings = OpenAIEmbeddings(openai_api_key='YOUR_OPENAI_API_KEY')
instance = Chroma(embedding_function=embeddings, persist_directory='PATH_TO_PERSIST_DIRECTORY')
loader = WebBaseLoader(tgt_sites)
if loader:
add_documents(loader, instance)
instance.persist()
instance = None
instance = Chroma(persist_directory='PATH_TO_PERSIST_DIRECTORY', embedding_function=embeddings)
Generating System Prompt Phrases
Now that we have set up our environment and loaded the necessary data, we can proceed to generate system prompt phrases using ChatGPT. We will utilize the RetrievalQA class from Langchain, which incorporates the ChatOpenAI model to interact with the language model. Here is the code snippet to generate system prompt phrases:
qa = RetrievalQA.from_chain_type(
llm=ChatOpenAI(
model_name="gpt-3.5-turbo",
temperature=0,
openai_api_key='YOUR_OPENAI_API_KEY'
),
chain_type="stuff",
retriever=instance.as_retriever()
)
query_str = """
Craft a paragraph of how chatgpt (address as you) supposed to act based on the role stated.
Provide expectation of the required scope, skillset and knowledge.
If there is no specific role found, use relative reference if necessary.
The role is "python blog professional writer". Maximium 5 sentences.
Start the paragraph with "I want you to act as a "
"""
output_string = qa.run(query_str)
print(output_string)
[Sample output]
I want you to act as a Python blog professional writer. As a ChatGPT, you are expected to have a good understanding of Python programming language and its various libraries and frameworks. You should be able to write informative and engaging blog posts that cater to both beginners and advanced users. Your writing should be clear, concise, and well-structured, with a focus on providing practical examples and use cases.Additionally, you should be able to keep up with the latest trends and developments in the Python community and incorporate them into your writing.
In this script, our focus is on generating system role prompts. You can append your specific requests or target tasks to these prompts for ChatGPT to understand and respond accordingly. To enhance ChatGPT’s capabilities, you can include additional relevant websites, expanding its knowledge base for prompt generation in various roles and scenarios.
Conclusion
In this blog post, we explored the process of using ChatGPT and additional data extracted from a webpage to generate system prompt phrases. By leveraging the power of language models and retrieval techniques, we can create informative and context-aware prompts to guide AI systems more efficiently.
This post has also been published on Medium.